Algorithms that I Learned
Mar 22, 2020
·
1 min read
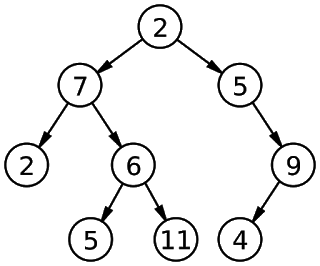
This is just a personal list of algorithms that I have learned over time. Since I keep on forgetting them, I decided I should make a list and review them from time to time. If you find the list helpful, thank me in the comments below.
Sorting
- Insertion Sort
- Selection Sort
- Bubble Sort
- Quick Sort
- Merge Sort
- Radix Sort
- Heap Sort
- Bogo Sort & Tim Sort 😛
Other things to know about sorting:
- Stable vs Unstable sort
Graph Searching and Shortest Path algorithms
- Linear Search
- Binary Search
- A* Search Algorithm
- Breadth First Search (BFS)
- Depth First Search (DFS)
- Minimum Spanning Tree
- Kruskal’s
- Prim’s
- Bellman Ford
- Dijkstra
- Floyd-Warshall
- Ford-Fulkerson algorithm (Flow Networks)
Greedy algorithms
- Fractional Knapsack Problem
- Activity Selection Problem
- Huffman Encoding
Pattern Matching Algorithms
- KMP
- Boyer-Moore
Dynamic programming
- Coin Changing Problem
- 0-1 Knapsack Problem
Other algorithms
- Apriori algorithm
- Matrix Chain Multiplication
- Simplex Algorithm and Integer Linear Programming
- Hashing (SHA-512)
- Prefix, Infix and Postfix Expressions
- Floyd’s Algorithm (The Tortoise and the Hare)
Other things to know:
- Static vs Dynamic Hashing
Data structures
- Stack
- Queue
- Linked Lists
- Singly Linked Lists
- Two way
- Circular Linked Lists
- Trees
- Binary search tree
- AVL tree (self-balancing binary tree)
- B Tree / B+ Tree
- Red-Black Tree
- N-ary tree
- Ukkonen’s algorithm (Suffix tree)
- Trie
- Heap
Sharing is caring!